HTML (Hypertext Markup Language) is the standard markup language used for creating web pages. An HTML document is a text file that contains the structure and content of a web page. It consists of a series of HTML tags, which are used to define the elements and attributes of the document.
The Document Object Model (DOM) is a programming interface for HTML and XML documents. It represents the structure of an HTML document as a tree-like structure, where each node in the tree represents an element or attribute in the document. The DOM allows developers to access, manipulate, and update the content and structure of an HTML document using programming languages such as JavaScript.
Understanding the DOM is crucial for web development because it allows developers to dynamically update and modify the content of a web page. By manipulating the DOM, developers can create interactive and dynamic web applications that respond to user input and update in real-time. Without a solid understanding of the DOM, it would be challenging to create complex and interactive web applications.
The Structure of an HTML Document: Tags, Elements, and Attributes
HTML documents are made up of tags, elements, and attributes. Tags are used to define the structure and content of an HTML document. They are enclosed in angle brackets (<>) and come in pairs – an opening tag and a closing tag. Elements are created by enclosing content within tags. For example, the
tag is used to define a paragraph element.
Attributes provide additional information about an element. They are added to the opening tag of an element and consist of a name-value pair. For example, the tag has attributes such as src (which specifies the source URL of the image) and alt (which provides alternative text for screen readers).
There are many different types of HTML tags that serve different purposes. Some common tags include:
–
to
: Used to define headings of different levels.
–
–
: Used to define paragraphs.
– : Used to create hyperlinks.
– : Used to insert images.
–
– : Used to apply styles to a specific section of text.
These are just a few examples of the many tags available in HTML. Each tag has its own set of attributes and can be used in different ways to create the desired structure and content of a web page.
Understanding the Document Object Model (DOM)
The Document Object Model (DOM) is a programming interface that represents an HTML document as a tree-like structure. Each node in the tree represents an element, attribute, or piece of text in the document. The DOM allows developers to access, manipulate, and update the content and structure of an HTML document using programming languages such as JavaScript.
The DOM tree starts with the root node, which represents the entire HTML document. From there, each element in the document is represented by a node in the tree. The relationship between elements is represented by parent-child relationships between nodes. For example, if an
element is nested inside a
element, the
element would be the parent node and the
element would be the child node.
Attributes are also represented as nodes in the DOM tree. They are attached to their corresponding element nodes and can be accessed and manipulated using DOM methods.
The Role of the DOM in HTML Document Rendering
When a web browser receives an HTML document, it uses the DOM to parse and render the document. The browser creates a DOM tree based on the structure and content of the HTML document. This DOM tree is then used by the browser’s rendering engine to display the web page on the screen.
A well-structured DOM is essential for efficient rendering. When the browser encounters invalid or poorly structured HTML, it may have to make assumptions or correct errors in order to create a valid DOM tree. This can lead to unexpected rendering issues and slower page load times.
By understanding the DOM and how it is used by browsers, developers can ensure that their HTML documents are well-structured and optimized for efficient rendering. This includes using valid HTML, avoiding unnecessary nesting of elements, and minimizing the use of inline styles and scripts.
How Browsers Interpret HTML Documents Using the DOM
When a browser receives an HTML document, it goes through a process called parsing. During parsing, the browser reads the document from top to bottom and creates a DOM tree based on the structure and content of the document.
The parsing process involves several steps. First, the browser tokenizes the document, breaking it down into individual tokens such as opening tags, closing tags, attributes, and text. It then constructs a parse tree based on these tokens, which represents the structure of the document.
As the parse tree is constructed, the browser also creates the corresponding DOM tree. Each element in the parse tree is converted into a node in the DOM tree. The DOM tree is then used by the browser’s rendering engine to display the web page on the screen.
If the browser encounters invalid HTML during parsing, it may have to make assumptions or correct errors in order to create a valid DOM tree. This can lead to unexpected rendering issues and slower page load times. It is therefore important to write valid and well-structured HTML to ensure that the browser can create an accurate representation of the document using the DOM.
The Hierarchy of Nodes in the DOM Tree
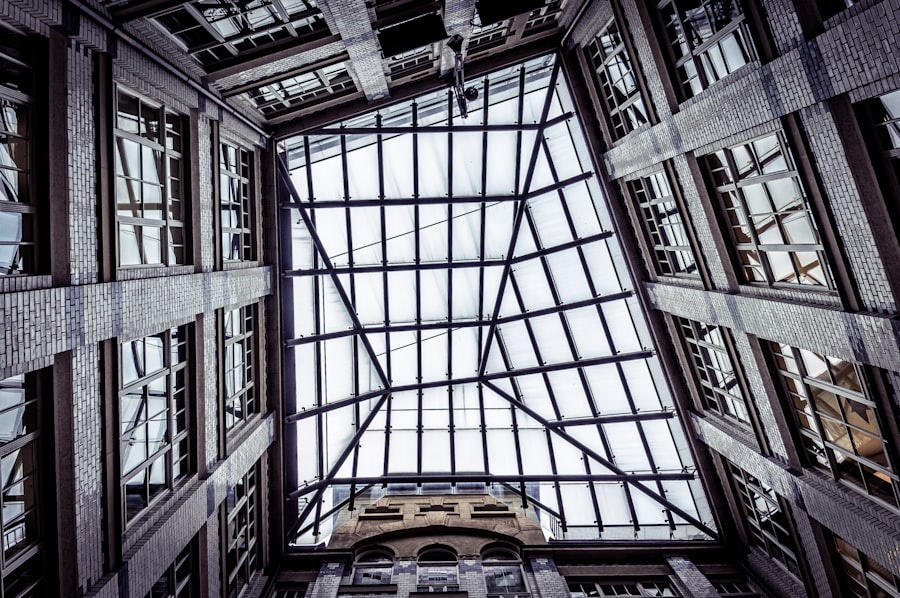
The DOM tree is organized in a hierarchical structure, with each node representing an element, attribute, or piece of text in the HTML document. There are several different types of nodes in the DOM tree, each with its own properties and methods.
The root node represents the entire HTML document and is the starting point of the DOM tree. It has child nodes that represent the different sections and elements of the document.
Element nodes represent HTML elements and are the most common type of node in the DOM tree. They have properties and methods that allow developers to access and manipulate their attributes, content, and structure.
Text nodes represent the text content of an element. They are child nodes of element nodes and contain the actual text that is displayed on the web page.
Attribute nodes represent the attributes of an element. They are attached to their corresponding element nodes and can be accessed and manipulated using DOM methods.
Comment nodes represent HTML comments and are used to add notes or annotations to the HTML code. They are not displayed on the web page but can be accessed and manipulated using DOM methods.
Document nodes represent the entire HTML document and are the parent node of the root node. They have properties and methods that allow developers to access and manipulate the entire document.
Manipulating HTML Documents with the DOM API
The DOM API (Application Programming Interface) provides a set of methods and properties for manipulating HTML documents using programming languages such as JavaScript. These methods allow developers to access, create, modify, and delete elements, attributes, and text in an HTML document.
Some common DOM API methods include:
– getElementById(): Retrieves an element from the DOM tree based on its unique ID.
– getElementsByClassName(): Retrieves a collection of elements from the DOM tree based on their class name.
– getElementsByTagName(): Retrieves a collection of elements from the DOM tree based on their tag name.
– createElement(): Creates a new element node in the DOM tree.
– appendChild(): Adds a new child node to an existing element node.
– removeChild(): Removes a child node from an existing element node.
– setAttribute(): Sets the value of an attribute for an existing element node.
– innerHTML: Gets or sets the HTML content of an element node.
These are just a few examples of the many methods available in the DOM API. By using these methods, developers can dynamically update and modify the content and structure of an HTML document, creating interactive and dynamic web applications.
Using JavaScript to Access and Modify the DOM
JavaScript is a programming language that can be used to interact with the DOM and manipulate HTML documents. It provides a powerful set of tools and methods for accessing, modifying, and updating the content and structure of an HTML document.
To access elements in the DOM using JavaScript, developers can use methods such as getElementById(), getElementsByClassName(), and getElementsByTagName(). These methods allow developers to retrieve elements from the DOM tree based on their ID, class name, or tag name.
Once an element is retrieved, developers can use JavaScript to modify its attributes, content, and structure. For example, they can use the setAttribute() method to change the value of an attribute, or the innerHTML property to update the content of an element.
JavaScript can also be used to create new elements and add them to the DOM tree. The createElement() method allows developers to create a new element node, which can then be added to an existing element using the appendChild() method.
By combining JavaScript with the DOM API, developers have a powerful toolset for creating dynamic and interactive web applications that respond to user input and update in real-time.
Best Practices for Working with the DOM
When working with the DOM, there are several best practices that developers should follow to optimize performance and write efficient and maintainable code.
One best practice is to minimize DOM manipulation. Manipulating the DOM can be a costly operation in terms of performance, especially when dealing with large or complex documents. It is therefore important to minimize unnecessary DOM manipulations and batch them together when possible.
Another best practice is to cache DOM references. Accessing elements in the DOM can be an expensive operation, especially when using methods such as getElementById() or getElementsByClassName(). By caching references to frequently accessed elements, developers can avoid unnecessary DOM traversals and improve performance.
It is also important to write valid and well-structured HTML. Invalid or poorly structured HTML can lead to unexpected rendering issues and slower page load times. By writing valid HTML, developers can ensure that the browser can create an accurate representation of the document using the DOM.
Finally, it is important to test and optimize DOM performance. Tools such as the Chrome DevTools can be used to analyze and profile DOM performance, allowing developers to identify and fix performance bottlenecks.
By following these best practices, developers can write efficient and maintainable code that interacts with the DOM, resulting in faster page load times and a better user experience.
Mastering the Anatomy of an HTML Document with the DOM
In conclusion, understanding the Document Object Model (DOM) is crucial for web development success. The DOM represents the structure of an HTML document as a tree-like structure, allowing developers to access, manipulate, and update the content and structure of a web page.
By understanding the structure of an HTML document, including tags, elements, and attributes, developers can create well-structured and valid HTML that can be accurately represented by the DOM.
The DOM plays a crucial role in how browsers interpret and render HTML documents. By understanding how browsers parse HTML documents and create the DOM tree, developers can optimize their code for efficient rendering.
Using the DOM API and JavaScript, developers can manipulate HTML documents dynamically, creating interactive and dynamic web applications. By following best practices for working with the DOM, developers can optimize performance and write efficient and maintainable code.
In conclusion, mastering the anatomy of an HTML document with the DOM is essential for web development success. By understanding how HTML documents are structured and how they are represented by the DOM, developers can create powerful and interactive web applications that provide a great user experience.
If you’re interested in learning more about the fascinating world of filmmaking, you might want to check out this article on The History of Filmmaking. It delves into the evolution of this art form, from its humble beginnings to the modern-day techniques used in the industry. Understanding the history of filmmaking can provide valuable insights and inspiration for aspiring filmmakers. So, if you’re ready to dive into the rich heritage of cinema, this article is a must-read.
FAQs
What is an HTML document?
An HTML document is a file that contains structured content and markup tags that define the structure and appearance of a web page.
What is the Document Object Model (DOM)?
The Document Object Model (DOM) is a programming interface for HTML and XML documents. It represents the page so that programs can change the document structure, style, and content.
What is the structure of an HTML document?
An HTML document consists of several parts, including the doctype declaration, the head section, and the body section. The doctype declaration specifies the version of HTML being used, while the head section contains metadata and links to external resources. The body section contains the actual content of the page.
What is the role of HTML tags in a document?
HTML tags are used to define the structure and content of a web page. They provide a way to mark up text, images, and other elements so that web browsers can interpret and display them correctly.
What is the difference between HTML and the DOM?
HTML is a markup language used to define the structure and content of a web page, while the DOM is a programming interface that represents the page as a tree-like structure of objects. The DOM provides a way for programs to interact with and manipulate the HTML document.
How does the DOM tree represent an HTML document?
The DOM tree represents an HTML document as a hierarchical structure of objects. The root of the tree is the document object, which contains the entire document. Each HTML element is represented by an element object, which contains properties and methods for manipulating the element and its contents.
element would be the child node.
Attributes are also represented as nodes in the DOM tree. They are attached to their corresponding element nodes and can be accessed and manipulated using DOM methods.
The Role of the DOM in HTML Document Rendering
When a web browser receives an HTML document, it uses the DOM to parse and render the document. The browser creates a DOM tree based on the structure and content of the HTML document. This DOM tree is then used by the browser’s rendering engine to display the web page on the screen.
A well-structured DOM is essential for efficient rendering. When the browser encounters invalid or poorly structured HTML, it may have to make assumptions or correct errors in order to create a valid DOM tree. This can lead to unexpected rendering issues and slower page load times.
By understanding the DOM and how it is used by browsers, developers can ensure that their HTML documents are well-structured and optimized for efficient rendering. This includes using valid HTML, avoiding unnecessary nesting of elements, and minimizing the use of inline styles and scripts.
How Browsers Interpret HTML Documents Using the DOM
When a browser receives an HTML document, it goes through a process called parsing. During parsing, the browser reads the document from top to bottom and creates a DOM tree based on the structure and content of the document.
The parsing process involves several steps. First, the browser tokenizes the document, breaking it down into individual tokens such as opening tags, closing tags, attributes, and text. It then constructs a parse tree based on these tokens, which represents the structure of the document.
As the parse tree is constructed, the browser also creates the corresponding DOM tree. Each element in the parse tree is converted into a node in the DOM tree. The DOM tree is then used by the browser’s rendering engine to display the web page on the screen.
If the browser encounters invalid HTML during parsing, it may have to make assumptions or correct errors in order to create a valid DOM tree. This can lead to unexpected rendering issues and slower page load times. It is therefore important to write valid and well-structured HTML to ensure that the browser can create an accurate representation of the document using the DOM.
The Hierarchy of Nodes in the DOM Tree
The DOM tree is organized in a hierarchical structure, with each node representing an element, attribute, or piece of text in the HTML document. There are several different types of nodes in the DOM tree, each with its own properties and methods.
The root node represents the entire HTML document and is the starting point of the DOM tree. It has child nodes that represent the different sections and elements of the document.
Element nodes represent HTML elements and are the most common type of node in the DOM tree. They have properties and methods that allow developers to access and manipulate their attributes, content, and structure.
Text nodes represent the text content of an element. They are child nodes of element nodes and contain the actual text that is displayed on the web page.
Attribute nodes represent the attributes of an element. They are attached to their corresponding element nodes and can be accessed and manipulated using DOM methods.
Comment nodes represent HTML comments and are used to add notes or annotations to the HTML code. They are not displayed on the web page but can be accessed and manipulated using DOM methods.
Document nodes represent the entire HTML document and are the parent node of the root node. They have properties and methods that allow developers to access and manipulate the entire document.
Manipulating HTML Documents with the DOM API
The DOM API (Application Programming Interface) provides a set of methods and properties for manipulating HTML documents using programming languages such as JavaScript. These methods allow developers to access, create, modify, and delete elements, attributes, and text in an HTML document.
Some common DOM API methods include:
– getElementById(): Retrieves an element from the DOM tree based on its unique ID.
– getElementsByClassName(): Retrieves a collection of elements from the DOM tree based on their class name.
– getElementsByTagName(): Retrieves a collection of elements from the DOM tree based on their tag name.
– createElement(): Creates a new element node in the DOM tree.
– appendChild(): Adds a new child node to an existing element node.
– removeChild(): Removes a child node from an existing element node.
– setAttribute(): Sets the value of an attribute for an existing element node.
– innerHTML: Gets or sets the HTML content of an element node.
These are just a few examples of the many methods available in the DOM API. By using these methods, developers can dynamically update and modify the content and structure of an HTML document, creating interactive and dynamic web applications.
Using JavaScript to Access and Modify the DOM
JavaScript is a programming language that can be used to interact with the DOM and manipulate HTML documents. It provides a powerful set of tools and methods for accessing, modifying, and updating the content and structure of an HTML document.
To access elements in the DOM using JavaScript, developers can use methods such as getElementById(), getElementsByClassName(), and getElementsByTagName(). These methods allow developers to retrieve elements from the DOM tree based on their ID, class name, or tag name.
Once an element is retrieved, developers can use JavaScript to modify its attributes, content, and structure. For example, they can use the setAttribute() method to change the value of an attribute, or the innerHTML property to update the content of an element.
JavaScript can also be used to create new elements and add them to the DOM tree. The createElement() method allows developers to create a new element node, which can then be added to an existing element using the appendChild() method.
By combining JavaScript with the DOM API, developers have a powerful toolset for creating dynamic and interactive web applications that respond to user input and update in real-time.
Best Practices for Working with the DOM
When working with the DOM, there are several best practices that developers should follow to optimize performance and write efficient and maintainable code.
One best practice is to minimize DOM manipulation. Manipulating the DOM can be a costly operation in terms of performance, especially when dealing with large or complex documents. It is therefore important to minimize unnecessary DOM manipulations and batch them together when possible.
Another best practice is to cache DOM references. Accessing elements in the DOM can be an expensive operation, especially when using methods such as getElementById() or getElementsByClassName(). By caching references to frequently accessed elements, developers can avoid unnecessary DOM traversals and improve performance.
It is also important to write valid and well-structured HTML. Invalid or poorly structured HTML can lead to unexpected rendering issues and slower page load times. By writing valid HTML, developers can ensure that the browser can create an accurate representation of the document using the DOM.
Finally, it is important to test and optimize DOM performance. Tools such as the Chrome DevTools can be used to analyze and profile DOM performance, allowing developers to identify and fix performance bottlenecks.
By following these best practices, developers can write efficient and maintainable code that interacts with the DOM, resulting in faster page load times and a better user experience.
Mastering the Anatomy of an HTML Document with the DOM
In conclusion, understanding the Document Object Model (DOM) is crucial for web development success. The DOM represents the structure of an HTML document as a tree-like structure, allowing developers to access, manipulate, and update the content and structure of a web page.
By understanding the structure of an HTML document, including tags, elements, and attributes, developers can create well-structured and valid HTML that can be accurately represented by the DOM.
The DOM plays a crucial role in how browsers interpret and render HTML documents. By understanding how browsers parse HTML documents and create the DOM tree, developers can optimize their code for efficient rendering.
Using the DOM API and JavaScript, developers can manipulate HTML documents dynamically, creating interactive and dynamic web applications. By following best practices for working with the DOM, developers can optimize performance and write efficient and maintainable code.
In conclusion, mastering the anatomy of an HTML document with the DOM is essential for web development success. By understanding how HTML documents are structured and how they are represented by the DOM, developers can create powerful and interactive web applications that provide a great user experience.
If you’re interested in learning more about the fascinating world of filmmaking, you might want to check out this article on The History of Filmmaking. It delves into the evolution of this art form, from its humble beginnings to the modern-day techniques used in the industry. Understanding the history of filmmaking can provide valuable insights and inspiration for aspiring filmmakers. So, if you’re ready to dive into the rich heritage of cinema, this article is a must-read.
FAQs
What is an HTML document?
An HTML document is a file that contains structured content and markup tags that define the structure and appearance of a web page.
What is the Document Object Model (DOM)?
The Document Object Model (DOM) is a programming interface for HTML and XML documents. It represents the page so that programs can change the document structure, style, and content.
What is the structure of an HTML document?
An HTML document consists of several parts, including the doctype declaration, the head section, and the body section. The doctype declaration specifies the version of HTML being used, while the head section contains metadata and links to external resources. The body section contains the actual content of the page.
What is the role of HTML tags in a document?
HTML tags are used to define the structure and content of a web page. They provide a way to mark up text, images, and other elements so that web browsers can interpret and display them correctly.
What is the difference between HTML and the DOM?
HTML is a markup language used to define the structure and content of a web page, while the DOM is a programming interface that represents the page as a tree-like structure of objects. The DOM provides a way for programs to interact with and manipulate the HTML document.
How does the DOM tree represent an HTML document?
The DOM tree represents an HTML document as a hierarchical structure of objects. The root of the tree is the document object, which contains the entire document. Each HTML element is represented by an element object, which contains properties and methods for manipulating the element and its contents.